The Laws of Motion
We already know how to move objects around using repeat and changing the location each time around the loop. But we need to know which changes to make so that the motion looks like that of a cannonball flying in the Earth's gravity. Isaac Newton was the first to write the laws of motion in mathematically correct form in 1687. His laws were so close to reality that it was only in 1905, more than two hundred years later, that Albert Einstein found laws that were closer to observation. But Newton's laws are so good that in daily life they are more than good enough for everything from cannonballs to putting a person on the Moon. We will therefore use them here.
Newton's laws of motion are very simple:
- The position of an object changes proportionally to its speed.
- The speed of an object changes proportionally to its acceleration.
- The acceleration of an object is proportional to the force that works on it and inversely proportional to its mass.
In plain language:
Acceleration is greater if the force is greater.
With the same force it is easier to kick a light object than a massive one.
The more acceleration, the faster the speed grows.
The higher the speed, the faster the position changes.
To understand this better, think of sitting in a car that stands still. Then you press the accelerator pedal hard: the motor produces a force on the car. That gives it the acceleration. But you were also not moving: the back of the seat which is connected to the car, has to push you hard to make you go forward. As you keep the pedal pressed, the speed of the car is going up and up and up. And of course your position is changing faster and faster. The same thing happens when you brake: the brakes produce a force but it is backwards, the acceleration is now directed towards the back, the seat belt forces you back, your speed changes with the acceleration, but because it is negative your speed decreases. And your position changes less and less fast. Until you stop.
Units
This section can be skipped since it is only important if you want to make a scaled simulation. In a scaled simulation objects behave like in real life and you could make measurements such as how long it takes for the cannonball to land etc. We will not do that in the cannonball program.
For formulae we want short, single letter names instead of using the full words speed, time, etc. The standard usage is s for location (Latin situ), v for speed (velocity), t for time, a for acceleration, m for mass and F for force. Some of these same letters are also used for the units! So do not confuse the m for mass with the m for metre!
Position or location s is measured in metres, abbreviated m.
Time t is measured in seconds, abbreviated s.
Speed v is the amount of position change in each second. Position is measured in metres, therefore speed is measured in metres per second or m/s. That is a real division: metres divided by seconds. A speed of 1 m/s? How much is that? There are 60 seconds in a minute and 60 minutes in an hour. There are 60*60=3600 seconds in an hour. If you do 1 metre each second, you do 3600 metres in an hour, or 3.6 km. 1 m/s = 3.6 km/h (walking speed).
Acceleration a is the amount of speed change in each second. Speed is measured in m/s, therefore acceleration is measured in (m/s)/s or m/s2. This may seem strange, but think again of sitting in a car. You are on a busy route leaving the city and drive at a constant speed of 10 m/s (that is 36 km/h). Then you come to the motorway, and you accelerate to 20 m/s (72 km/h) because there is less traffic now. If it takes you 10 seconds to increase your speed from 10 m/s to 20 m/s, then you have added 1 m/s to your speed for every second that you were accelerating. You have accelerated at 1 m/s per second, or 1 m/s/s or 1 m/s2. Think it over again because you went too fast.
Mass m is measured in kilograms or kg.
Force F is what causes the acceleration. Acceleration is bigger if the force is bigger, and it is smaller if the mass of the thing you push is larger. It's then easy to see that a = F/m . Or: F = a*m (we use the asterisk * for multiplication, because that is what programming languages use to avoid confusion with the letter x.) This means that F is measured in kg*m/s2. I won't go any further than this and we will actually not use units in our program.
Doing the simulations
Let us look at an object that goes along a line, a railway car on a railroad for example.
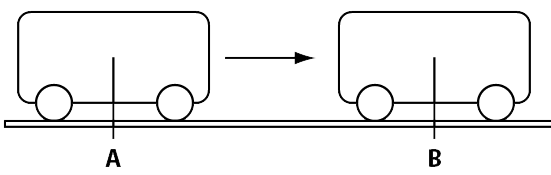
It starts at place A and moves along at constant speed to place B. If we had to make a movie of that motion, we would have to show many images per second. Remember the moving object in the Ball stack of the previous page: in each image the railroad car would be a little bit further along than in the previous image.
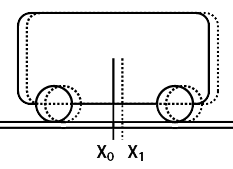
The difference would be proportional to the speed: the new position X1 (eks-one) would be equal to the old position X0 (eks-zero) plus something times the speed:
X1 = X0 + V*k1
The number k1 depends on the images per second and whether we want to make the film realistic, speed it up or slow it down. It's not important what k1 is, as long as the film looks reasonable. Choosing the right value for k1 is important if we make a scaled simulation, but we have decided not to do that.
It's the numbers like X1 and X0 that we will use in setting the loc of an object.
Now let's look at V: if there is an acceleration A then V also changes from one image to the next of our movie:
V1 = V0 + A*k2
and k2 is a different number from k1, but it has a similar function.
Now A itself is rather easy: we're going to keep it fixed at some value.
The simulation loop
We can write a bit of program to generate the images of our movie. You could pick up the Motion.rev file again and change the script of the Move button to:
on MouseUp
send "MouseUp" to button "Reset"
put the loc of graphic "Ball" into lBallLocation
put item 1 of lBallLocation into lX
put item 2 of lBallLocation into lY
put 0 into lV
put 1 into lAcceleration
put 1 into lK1
put 1 into lK2
repeat 30 times
set the loc of graphic "Ball" to lX,lY
add lAcceleration*lK2 to lV
add lV*lK1 to lX
wait for 2 ticks
end repeat
end MouseUp
Before you press the Move button, a few notes on the Transcript language used here:
I have again applied my rule that local variables start with the lowercase letter l and then have a capital letter. I have used the same symbols k1, k2 etc. as in the explanation above, I just started with a capital letter.
Any text after "--" is a comment.
I have used whole numbers for lK1, lK2 and lAcceleration and we will soon see why.
Now make the program run by selecting the browse tool and press the Move button. The ball starts relatively slowly, then accelerates and moves even out of the window! (fortunately we have the Reset button).
Getting a better understanding
If you are really intelligent and quick, you may have found this all boring, but if you are like me then it's a good exercise to do the calculations by hand to see what's really happening:
time around the repeat loop |
Acceleration |
Speed V |
Position X |
0 |
1 |
0 |
40 |
1 |
1 |
1 |
41 |
2 |
1 |
2 |
43 |
3 |
1 |
3 |
46 |
4 |
1 |
4 |
50 |
5 |
1 |
5 |
55 |
... |
1 |
... |
... |
Each time we go around the repeat we add the acceleration to the speed and then that new speed to the position. We see that the acceleration is constant. The speed increases in steps of 1. The position increases in steps that grow, because the speed grows.
We forgot an important fact about locations
Locations are always in pixels. It is impossible to place something on the screen "in between pixels". The two numbers of the loc of an object must be whole numbers!
Suppose things look better if we set the acceleration ay to 0.5 instead of 1, and use 2.34 for k1 and k2?
Then all our calculations may be better, but we will not have whole numbers for x and y. Revolution will truncate the values to whole numbers. If the successive values for position x would be 41.0 41.3 41.6 41.9 42.2 ... then Revolution would set the loc in x successively to 41 41 41 41 42 ... because it would simply throw away the digits after the decimal point.
That's not so good: things would look smoother if it would round the values and use 41 41 42 42 42 ... instead.
Therefore, we should change the statement
set the loc of graphic "Ball" to lX,lY
to
set the loc of graphic "Ball" to round(lX),round(lY)
Running the Debugger
We can see what happens by switching on the debugger.
But let's do that on the next issue.
|